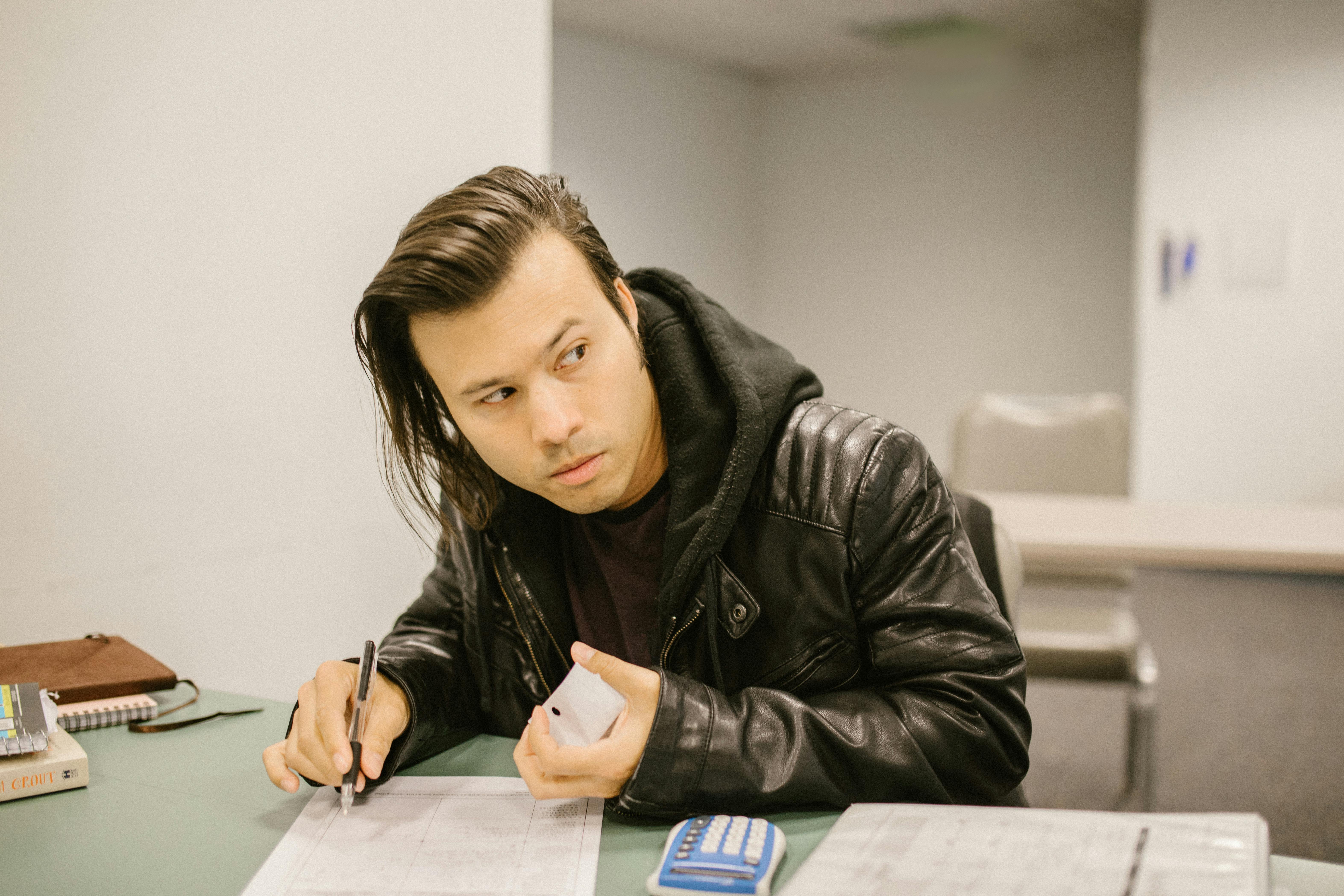
How to Effectively Reverse a List in Python
Reversing a list is a fundamental task in Python programming that can enhance your coding skills significantly. As lists are one of the primary data structures used in Python, understanding how to manipulate them, specifically reversing their order, is crucial. Whether you're a beginner learning Python or an advanced developer optimizing algorithms, mastering list reversal will benefit your programming journey.
This article will explore various methods to reverse a list in Python, detailing the Python reverse method, slicing techniques, loops, and even recursion. By following the guide, you will discover efficient list manipulation techniques that can solve common programming problems effectively.
Here’s what to expect in this article:
- Different ways to reverse a list in Python
- Practical examples and coding snippets
- Best practices and optimization tips
Essential Python List Manipulation Techniques
Before diving into the specifics of how to reverse a list, it's important to understand the basics of Python lists. A Python list is an ordered collection of items that can contain different data types, making them versatile for various applications. Knowing how to manipulate lists efficiently is critical for any Python developer.
Understanding Python Lists Overview
Python lists are mutable, meaning their contents can be changed without creating a new list. This characteristic allows for a broad range of operations, including adding, removing, and reversing items. Understanding the properties of lists is vital for efficiently code writing.
Common Functions in Python for List Handling
Python offers numerous functions for handling lists, including:
- append()
- extend()
- insert()
- remove()
- pop()
These functions form the backbone of list operations, and knowing them helps in manipulating lists effectively.
Practical Use Cases for List Reversal
Reversing lists can serve various practical purposes, such as:
- Data analysis, where reversing the order of elements can provide a different perspective.
- Implementing algorithms that require reverse traversal of lists.
- Reversing strings and managing user inputs in applications.
Implementing these techniques makes your code not only effective but also efficient for data manipulation in Python.
Using Python Built-In Methods for List Reversal
Python provides several built-in methods for reversing lists. Let’s examine these methods to understand their efficiencies and how to implement them correctly.
Utilizing the Python Reverse Method
The simplest way to reverse a list in Python is by using the reverse()
method. This method reverses the list in place, which means it modifies the original list rather than creating a new one. Here's how it works:
my_list = [1, 2, 3, 4, 5]
my_list.reverse()
print(my_list) # Output: [5, 4, 3, 2, 1]
Implementing Slicing for List Reversal
Slicing is a powerful feature in Python that allows for concise and efficient list manipulations. To reverse a list using slicing, you can use the syntax list[::-1]
:
my_list = [1, 2, 3, 4, 5]
reversed_list = my_list[::-1]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Reversing a List with Python Loops
Another approach to reverse a list is by using loops. You can achieve this by iterating through the list and appending each element to a new list. Although this method is generally less efficient than using the reverse()
method or slicing, it provides great insight into list iteration:
my_list = [1, 2, 3, 4, 5]
reversed_list = []
for i in range(len(my_list) - 1, -1, -1):
reversed_list.append(my_list[i])
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Advanced Techniques for Reversing Lists in Python
For those looking to deepen their understanding of list manipulation in Python, several advanced techniques can help improve performance and functionality.
In-Place List Reversal Techniques
In-place list reversal is efficient because it doesn't require additional space for a new list. The reverse()
method is the most straightforward way to achieve this:
my_list = [1, 2, 3, 4, 5]
my_list.reverse() # List is now reversed in place
Reversing a List Using Recursion
Recursion is a powerful programming concept that can also be used to reverse a list. The idea is to swap the first element with the last and then recursively reverse the remaining elements:
def reverse_recursion(lst):
if len(lst) == 0:
return lst
return [lst[-1]] + reverse_recursion(lst[:-1])
my_list = [1, 2, 3, 4, 5]
print(reverse_recursion(my_list)) # Output: [5, 4, 3, 2, 1]
Reverse List Function: Creating Custom Python Solutions
Creating a custom function for reversing lists can be useful for understanding the mechanics behind list operations. Here’s an example:
def reverse_list(my_list):
return my_list[::-1]
my_list = [1, 2, 3, 4, 5]
print(reverse_list(my_list)) # Output: [5, 4, 3, 2, 1]
Common Pitfalls in List Reversal and Optimization Tips
While working with list reversals, specific common mistakes can affect your code's performance and functionality. Recognizing these pitfalls and incorporating optimization techniques can improve your programming skills significantly.
Avoiding List Copying Issues
One common issue is inadvertently creating copies of the list rather than modifying it in place. Always be mindful of which methods create a new list and which modify the original one.
Optimizing Your Reversal Algorithm
For large datasets, choose methods that minimize space complexity, such as in-place sorting algorithms. This practice helps with efficiency, especially in memory-constrained environments.
Testing and Validating List Output
It's essential to validate the output of your list reversal operations. Ensuring your reversed list matches expected results will prevent runtime errors and enhance debugging effectiveness. Use assertions to check your results during development.
Q&A: Addressing Common Questions on List Reversal
What is the best method to reverse a list in Python?
The best method depends on the use case; for most scenarios, the reverse()
method is efficient and simple. Slicing is also a quick option for creating a reversed copy without altering the original list.
Can I reverse a list while looping through it?
Yes, but ensure you create a new list to avoid modifying the list you're iterating over during the loop to prevent runtime errors.
Are there significant performance differences between these methods?
Yes, methods that create a new list like slicing can be less efficient in memory usage compared to in-place methods like reverse()
. For large lists, opting for in-place reversal is typically better.
How can I reverse a list of strings in Python?
You can use the same methods outlined above. For instance, using slicing: reversed_strings = my_string_list[::-1]
.
Where can I learn more Python list techniques?
Explore Python education resources and tutorials that focus on data manipulation in Python. Websites, coding boot camps, and books offer practical examples and comprehensive skills development.
By mastering these techniques and understanding list manipulations thoroughly, you'll enhance your Python programming skills significantly, enabling you to tackle more complex tasks in 2025 and beyond.